Create a free developer account
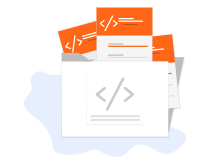
DOCUMENTATION
DOCUMENTATION
Access Developer Documentation and start transacting online.
View Documentation
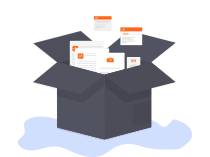
SDKs
SDKs
Get started quickly. Use our SDKs PHP, .NET, PYTHON and more.
Get SDKs
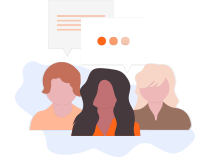
FORUM
FORUM
Join our community and help each other integrate and #GetPaid.
Browse Forums
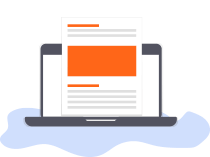
BLOG
BLOG
Get the latest Fintech news, information and tips.
View Blog
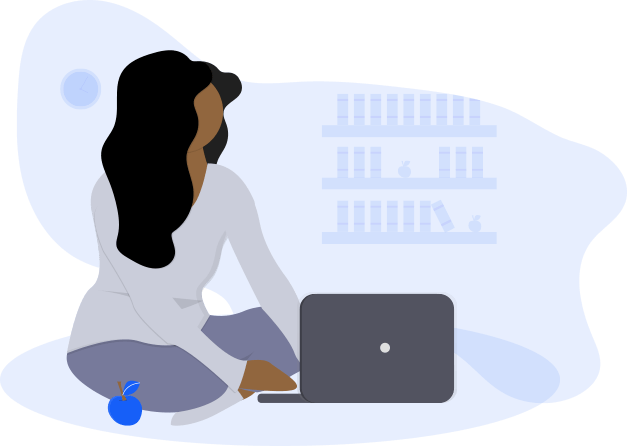
Getting Started
Obtain the Integration API keys
PaynowZW authenticates all API requests using your integration's API keys. You will need the API keys to successfully make requests to the API request.
You can create integrations and keys via the Dashboard as described here
- PHP
- .NET
- NodeJS
- Python
- Java
- Install Library
- Make an API request
- Handle API response
- Next Steps
This library has a set of prerequisites that must be met for it to work
- PHP version 5.6 or higher
- Curl extension
The library can be installed via Composer as shown below:
$ composer require paynow/php-sdk
and include the composer autoloader
<?php
require_once 'path/to/vendor/autoload.php';
// Do stuff
Alternatively, if you do not have composer installed, first download the library here. And include the autoloader file included with the library
<?php
require_once 'path/to/library/autoloader.php';
// Do stuff
$paynow = new Paynow\Payments\Paynow(
'INTEGRATION_ID',
'INTEGRATION_KEY',
'http://example.com/gateways/paynow/update', // This is the result URL
'http://example.com/return?gateway=paynow' // This is the return URL
);
// Create new payment and pass in the reference and payer's email address
$payment = $paynow->createPayment('Invoice 35', 'user@example.com');
// Add items and their amounts to the payment
$payment->add('Bananas', 2.50);
$payment->add('Apples', 3.40);
// Send the payment and save the response from Paynow in a variable
$response = $paynow->send($payment);
if($response->success()) {
// Get the link to redirect the user to, then use it as you see fit
$link = $response->redirectUrl();
// Get the poll url (used to check the status of a transaction).
// You might want to save this in your DB
$pollUrl = $response->pollUrl();
}
Congratulations! Read on to begin integrating Paynow: Full Guide
Alternatively, you can use any of the following plugins written for:
- Install Library
- Make an API request
- Handle API response
- Next Steps
This library has a set of prerequisites that must be met for it to work
- .NET 4.0 or later
To install via the Nuget package manager console, run the following command
PM> Install-Package Paynow
and import the library as shown below:
using Webdev.Payments.Paynow;
var paynow = new Paynow("INTEGRATION_ID", "INTEGRATION_KEY");
paynow.ResultUrl = "http://example.com/gateways/paynow/update";
paynow.ReturnUrl = "http://example.com/return?gateway=paynow";
// The return url can be set at later stages. You might want to do
// this if you want to pass data to the return url (like the reference
// of the transaction)
// Create new payment and pass in the reference and payer's email address
var payment = paynow.CreatePayment("Invoice 35");
// Passing in the name of the item and the price of the item
payment.add("Bananas", 2.5m);
payment.add("Apples", 3.4m);
// Save the response from paynow in a variable
var response = paynow.Send(payment);
var response = paynow.Send(payment);
if(response.Success())
{
// Get the url to redirect the user to so they can make payment
var link = response.RedirectLink();
// Get the poll url of the transaction
var pollUrl = response.PollUrl();
}
Congratulations! Read on to begin integrating Paynow: Full Guide
- Install Library
- Make an API request
- Handle API response
- Next Steps
This library has a set of prerequisites that must be met for it to work
- Node version 0.6.0 and above
- NPM (node's package manager, used to install the node library)
Install the library using NPM or yarn
$ npm install --save paynow
or
$ yarn add paynow
and import the library as shown below:
const Paynow = require("paynow");
let paynow = new Paynow("INTEGRATION_ID", "INTEGRATION_KEY");
paynow.resultUrl = "http://example.com/gateways/paynow/update";
paynow.returnUrl = "http://example.com/return?gateway=paynow";
// The return url can be set at later stages. You might want to do
// this if you want to pass data to the return url (like the reference
// of the transaction)
// Create new payment and pass in the reference and payer's email address
let payment = paynow.createPayment("Invoice 35");
// Passing in the name of the item and the price of the item
payment.add("Bananas", 2.5);
payment.add("Apples", 3.4);
// Save the response from paynow in a variable
let response = paynow.send(payment);
// Check if request was successful
if (response.success) {
// Get the poll url (used to check the status of a transaction). You might
// want to save this in your DB
let pollUrl = response.pollUrl;
// Get the instructions
let instructions = response.instructions;
} else {
// Ahhhhhhhhhhhhhhh
// *freak out*
}
Congratulations! Read on to begin integrating Paynow: Full Guide
- Install Library
- Make an API request
- Handle API response
- Next Steps
This library has a set of prerequisites that must be met for it to work
- Requests
- pip - a package manager for Python packages
$ pip install paynow
and import the Paynow class into your project
from paynow import Paynow
# Do stuff
paynow = Paynow(
'INTEGRATION_ID',
'INTEGRATION_KEY',
'http://example.com/gateways/paynow/update',
'http://example.com/return?gateway=paynow'
)
# Create new payment and pass in the reference and payer's email address
payment = paynow.create_payment('Order #100', 'test@example.com')
# Passing in the name of the item and the price of the item
payment.add('Bananas', 2.50)
payment.add('Apples', 3.40)
# Save the response from paynow in a variable
response = paynow.send(payment)
if response.success:
# Get the link to redirect the user to, then use it as you see fit
link = response.redirect_url
# Get the poll url (used to check the status of a transaction).
# You might want to save this in your DB
pollUrl = response.poll_url
Congratulations! Read on to begin integrating Paynow: Full Guide
- Install Library
- Make an API request
- Handle API response
- Next Steps
This library has a set of prerequisites that must be met for it to work
- Java JDK 7 or higher
Install the library using either Gradle:
repositories {
mavenCentral()
}
dependencies {
compile 'zw.co.paynow:java-sdk:1.1.1'
}
OR using Maven:
<dependency>
<groupId>zw.co.paynow</groupId>
<artifactId>java-sdk</artifactId>
<version>1.1.1</version>
</dependency>
and import the library into your project using the code below:
import webdev.core.*;
import webdev.payments.Paynow;
import webdev.payments.Payment;
Paynow paynow = new Paynow("INTEGRATION_ID", "INTEGRATION_KEY");
paynow.setResultUrl("http://example.com/gateways/paynow/update");
paynow.setReturnUrl("http://example.com/return?gateway=paynow");
// The return url can be set at later stages. You might want to do
// this if you want to pass data to the return url (like the reference
// of the transaction)
//
Payment payment = paynow.createPayment("Invoice 35");
// Passing in the name of the item and the price of the item
payment.add("Bananas", 2.5);
payment.add("Apples", 3.4);
// Save the response from paynow in a variable
WebInitResponse response = paynow.send(payment);
WebInitResponse response = paynow.send(payment);
if(response.success())
{
// Get the url to redirect the user to so they can make payment
String redirectUrl = response.redirectURL();
// Get the poll url of the transaction
String pollUrl = response.pollUrl();
}
Congratulations! Read on to begin integrating Paynow: Full Guide
Create a free developer account